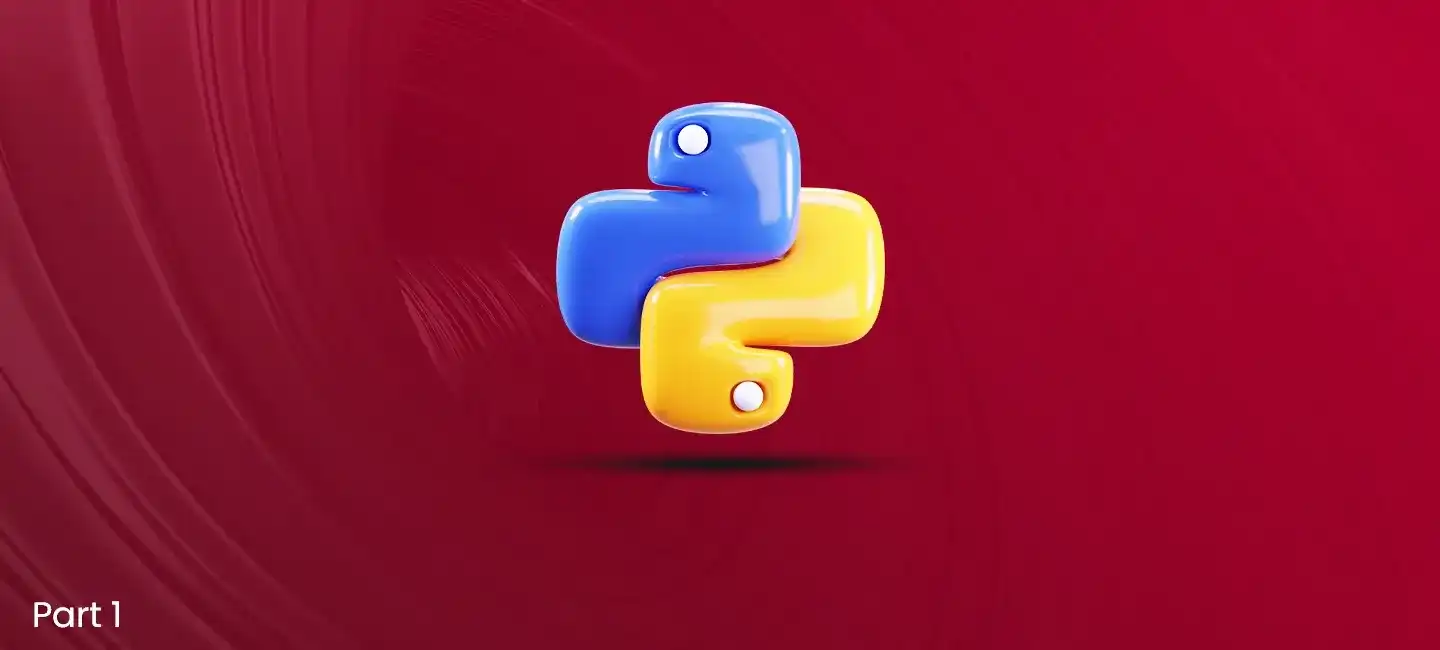
Blockchain Python Tutorial (Part-1) - An Ultimate Guide to Build a Simple Blockchain
Table of Contents
This article gives you a step-by-step guide on how to build a Blockchain in Python. In part 1, we’ll discuss the core functions of the blockchain network in detail and focus more on implementing them through programming.
What is a Blockchain?
A growing chain of blocks (records) that are linked together through strong cryptographic functions is known as a blockchain. It is a time-stamped decentralized network controlled by the participants of the network without any central authority. Bitcoin was the first application of a blockchain system after which many other cryptocurrency platforms development were founded on the same principles.
Fundamentally, blockchain has the following characteristics due to which businesses are opting to shift their entire business infrastructure to the blockchain network.
-
Decentralization
-
Immutability
-
Interoperability
-
Transparency
-
Security
All these qualities maintain the integrity of the blockchain network, ensure enhanced authenticity, and offer controlled access to the members. A lot of systems today rely on Blockchains such as NFT marketplaces, cryptocurrencies, and real-world industrial apps. It is worth noting that Blockchain does not have to be a complex program, instead, it is a list of transactions/records linked together.
In this tutorial, we’ll help you build a simple Python based blockchain from scratch. So, without any further delay, let’s get started.
How to Build a Blockchain in Python?
Here we will build a blockchain in Python by using an example of some basic cryptocurrency which we’ll call NeuralCoin (NC). NC will have some transactions as mentioned below.
T1: Anna sends bob 2 NC
T2: Bob sends Daniel 4.3 NC
T3: Mark sends Charlie 3.2 NC
These are some basic transactions that will be stored in blocks in the blockchain network. So suppose we have the first block B1. The first block of the blockchain network is called the ‘genesis block’. It will have all the information of the next three blocks/transactions but the genesis block does not have any additional hash information or we can say it has only some basic information that we can call ‘AAA’.
B1: (“AAA”, T1, T2, T3) -> 76fd89
Now this block will be hashed and it’ll result in a certain hash output. Normally, for hashing purposes, we use SHA 256 function. For your understanding of basic blockchain architecture, we can give some hash value to this block and the others. The hash value of one block is the basis of the next block. In this way, the connection and security of the blockchain are maintained.
B2: (“76fd89”, T4, T5, T6) -> 8923ff
This architecture of blockchain makes the network immutable because no entity could make changes in any block. Any change can cause a mess in the entire blockchain as we can see how dense transactions and blocks are interconnected.
So let’s move toward coding.
First, we’ll import an important Python blockchain library. The hashlib library is used to calculate a digital fingerprint for each block in the blockchain network.
import hashlib
class NeuralCoinBlock:
def __init__(self, previous_block_hash, transaction_list):
self.previous_block_hash = previous_block_hash
self.transaction_list = transaction_list
self.block_data = "-".join(transaction_list) + "-" + previous_block_hash
self.block_hash = hashlib.sha256(self.block_data.encode()).hexadigest()
The __init__ method stores a list of all the blocks in the blockchain. Here we have implemented the hashing of the block by concatenating all the transactions and the hash value of the previous block.
t1 = "Anna sends 2 NC to Mike"
t2 = "Bob sends 4.1 NC to Mike"
t3 = "Mike sends 3.2 NC to Bob"
t4 = "Daniel sends 0.3 NC to Anna"
t5 = "Mike sends 1 NC to Charlie"
t6 = "Mike sends 5.4 NC to Daniel"
initial_block - NeuralCoinBlock("Initial String", [t1, t2])
print (initial_block.block_data)
print(initial_block.block_hash)
This piece of code will first print the block data and then the hash of the first block. Now let's create the second and third blocks.
second_block = NeuralCoinBlock(initial_block.block_hash, [t3, t4])
print (second_block.block_data)
print(second_block.block_hash)
third_block = NeuralCoinBlock(second_block.block_hash, [t5, t6])
print (third_block.block_data)
print(third_block.block_hash)
Blockchain projects are in high demand due to their decentralized, secure, and immutable nature. So for instance, if you make a little change in the transaction value of any of the above transactions, the whole output of the network will change. The blockchain serves the purpose of transparency among all the network participants so that a democratized ecosystem could be built.
Hire a Python Developer!
Build foolproof Python blockchain projects with our agile and high-grade Python developers. Partner with InvoBlox’s well-versed team of Python full-stack developers who are committed to your project’s success from the ground up.
Hire a Python Developer
In the second part of this tutorial we will move towards some advanced concepts that will cover how:
-
To build a simple blockchain data structure
-
To add blocks in the blockchain
-
To validate the blockchain
Stay tuned!
In this tutorial, you’ve successfully learned the basic concepts of blockchain and built your first blockchain. You’ve learned how hashing works in the blockchain and integrity is maintained throughout the network to make sure the data remain unchanged and transparent for each participant.
Table of Contents
This article gives you a step-by-step guide on how to build a Blockchain in Python. In part 1, we’ll discuss the core functions of the blockchain network in detail and focus more on implementing them through programming.
What is a Blockchain?
A growing chain of blocks (records) that are linked together through strong cryptographic functions is known as a blockchain. It is a time-stamped decentralized network controlled by the participants of the network without any central authority. Bitcoin was the first application of a blockchain system after which many other cryptocurrency platforms development were founded on the same principles.
Fundamentally, blockchain has the following characteristics due to which businesses are opting to shift their entire business infrastructure to the blockchain network.
-
Decentralization
-
Immutability
-
Interoperability
-
Transparency
-
Security
All these qualities maintain the integrity of the blockchain network, ensure enhanced authenticity, and offer controlled access to the members. A lot of systems today rely on Blockchains such as NFT marketplaces, cryptocurrencies, and real-world industrial apps. It is worth noting that Blockchain does not have to be a complex program, instead, it is a list of transactions/records linked together.
In this tutorial, we’ll help you build a simple Python based blockchain from scratch. So, without any further delay, let’s get started.
How to Build a Blockchain in Python?
Here we will build a blockchain in Python by using an example of some basic cryptocurrency which we’ll call NeuralCoin (NC). NC will have some transactions as mentioned below.
T1: Anna sends bob 2 NC
T2: Bob sends Daniel 4.3 NC
T3: Mark sends Charlie 3.2 NC
These are some basic transactions that will be stored in blocks in the blockchain network. So suppose we have the first block B1. The first block of the blockchain network is called the ‘genesis block’. It will have all the information of the next three blocks/transactions but the genesis block does not have any additional hash information or we can say it has only some basic information that we can call ‘AAA’.
B1: (“AAA”, T1, T2, T3) -> 76fd89
Now this block will be hashed and it’ll result in a certain hash output. Normally, for hashing purposes, we use SHA 256 function. For your understanding of basic blockchain architecture, we can give some hash value to this block and the others. The hash value of one block is the basis of the next block. In this way, the connection and security of the blockchain are maintained.
B2: (“76fd89”, T4, T5, T6) -> 8923ff
This architecture of blockchain makes the network immutable because no entity could make changes in any block. Any change can cause a mess in the entire blockchain as we can see how dense transactions and blocks are interconnected.
So let’s move toward coding.
First, we’ll import an important Python blockchain library. The hashlib library is used to calculate a digital fingerprint for each block in the blockchain network.
import hashlib
class NeuralCoinBlock:
def __init__(self, previous_block_hash, transaction_list):
self.previous_block_hash = previous_block_hash
self.transaction_list = transaction_list
self.block_data = "-".join(transaction_list) + "-" + previous_block_hash
self.block_hash = hashlib.sha256(self.block_data.encode()).hexadigest()
The __init__ method stores a list of all the blocks in the blockchain. Here we have implemented the hashing of the block by concatenating all the transactions and the hash value of the previous block.
t1 = "Anna sends 2 NC to Mike"
t2 = "Bob sends 4.1 NC to Mike"
t3 = "Mike sends 3.2 NC to Bob"
t4 = "Daniel sends 0.3 NC to Anna"
t5 = "Mike sends 1 NC to Charlie"
t6 = "Mike sends 5.4 NC to Daniel"
initial_block - NeuralCoinBlock("Initial String", [t1, t2])
print (initial_block.block_data)
print(initial_block.block_hash)
This piece of code will first print the block data and then the hash of the first block. Now let's create the second and third blocks.
second_block = NeuralCoinBlock(initial_block.block_hash, [t3, t4])
print (second_block.block_data)
print(second_block.block_hash)
third_block = NeuralCoinBlock(second_block.block_hash, [t5, t6])
print (third_block.block_data)
print(third_block.block_hash)
Blockchain projects are in high demand due to their decentralized, secure, and immutable nature. So for instance, if you make a little change in the transaction value of any of the above transactions, the whole output of the network will change. The blockchain serves the purpose of transparency among all the network participants so that a democratized ecosystem could be built.
Hire a Python Developer!
Build foolproof Python blockchain projects with our agile and high-grade Python developers. Partner with InvoBlox’s well-versed team of Python full-stack developers who are committed to your project’s success from the ground up.
Hire a Python Developer
In the second part of this tutorial we will move towards some advanced concepts that will cover how:
-
To build a simple blockchain data structure
-
To add blocks in the blockchain
-
To validate the blockchain
Stay tuned!
In this tutorial, you’ve successfully learned the basic concepts of blockchain and built your first blockchain. You’ve learned how hashing works in the blockchain and integrity is maintained throughout the network to make sure the data remain unchanged and transparent for each participant.