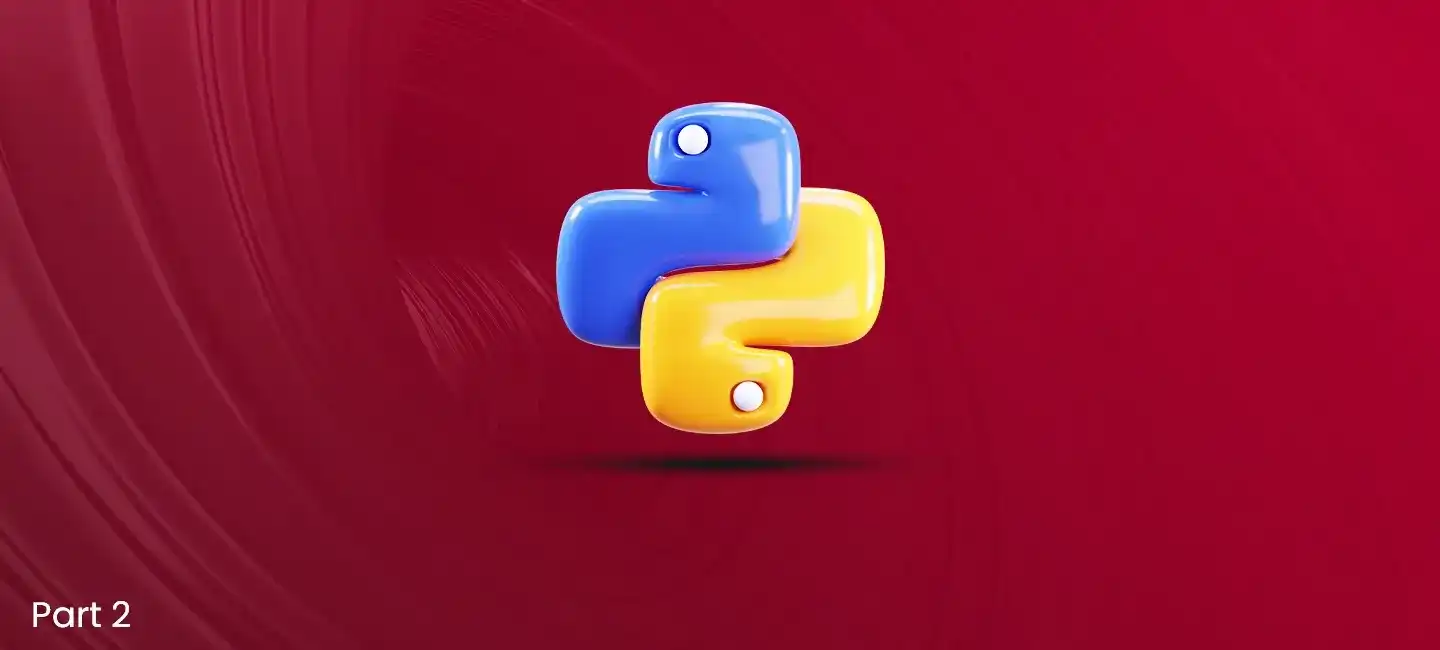
Blockchain Python Tutorial (Part-2) - An Ultimate Guide to Build a Simple Blockchain
Table of Contents
Blockchain Tutorial Python Part-1 explains the basics of blockchain technology and its implementation. In Part-2, we’ll move towards some advanced concepts of blockchain architecture and learn how to implement these components using the Python programming language.
Why is Python good for blockchain?
Python programming language is the best to choose for blockchain implementation if you are a beginner. The main reason is that Python has comparatively shortcodes and is easier to use.
Building the Blockchain
This article provides a step-by-step guide to building a simple Python blockchain. So let’s get started.
How to implement blockchain in Python?
-
Instead of setting up any complicated development environment, we’ll simply go to Colaboratory, where you can easily write and execute code in Python
-
All you need to do is go to the website, log in using your Google account, and start writing Python code
-
Go to file and open a new notebook tab
First, import the Python blockchain library and create a block class along with a constructor.
import hashlib
class Block:
def _init_(self, data, prev_hash):
self.data = data
self.prev_hash = prev_hash
self.hash = self.calc_hash()
Now we’ll create a method that calculates the hash. For this, Python can use any standard cryptographic hash technique as mentioned in the US National Security Agency’s (NSA) set of SHA functions. As mentioned in the previous part, one of the major characteristics of blockchain is immutability which can be implemented using cryptography. This is a one-way algorithm that hashes the data to ensure security and privacy in the blockchain network. Here we will implement SHA-256 to compute the hash of the block.
def calc_hash(self):
sha = hashlib.sha256()
sha.update(self.data.encode('utf-8'))
return sha.hexdigest()
Now let’s create a blockchain class. To ensure immutability in entire blockchain networks, the blocks are interconnected with each other in such a way that the hash of the previous block is within the next block. This is how blockchain implementation in Python maintains integrity in the blockchain.
Here we initialize the blockchain and create the first block of the blockchain which is also known as the ‘Genesis Block’.
class Blockchain:
def _init_(self):
self.chain = [self.create_genesis_block()]
def create_genesis_block(self):
return Block("Genesis Block", "0")
In the next step, we’ll write code to add new blocks to the blockchain. Each new block has the hash value of the previous block. In this way, the blocks in the blockchain are connected to each other ensuring data security and controlled access.
def add_block(self, data):
prev_block = self.chain[-1]
new_block = Block(data, prev_block.hash)
self.chain.append(new_block)
Now let’s test the blockchain we’ve built. The code below prints the hash value of the current block and previous block with which you can see if your blockchain is giving the right output or not.
Blockchain = Blockchain()
blockchain.add_block('First block')
blockchain.add_block('Second block')
blockchain.add_block('Third block')
print('Blockchain:")
for block in blockchain.chain:
print('Data:', block.data)
print('Previous hash:', block.prev_hash)
print('Hash:', block.hash)
print()
Congratulations! You just built a simple Blockchain in Python from scratch.
Hire a Python Developer!
Build foolproof Python blockchain projects with our agile and high-grade Python developers. Partner with InvoBlox’s well-versed team of Python full-stack developers who are committed to your project’s success from the ground up.
Hire a Python Developer
In Python blockchain development, you can implement more functions like consensus method (proof-of-work or any other to define rules by which enhanced security can be achieved) or mining to strengthen the immutability of your blockchain.
A proof is a random number which is hard to find unless you have a high performance machine and equipment in place that is running around the clock to compute values. Other than this, you can add other details such as fees/reward for miners to mine the block, public/private keys, etc. that scale-up the functionality of your blockchain.
Table of Contents
Blockchain Tutorial Python Part-1 explains the basics of blockchain technology and its implementation. In Part-2, we’ll move towards some advanced concepts of blockchain architecture and learn how to implement these components using the Python programming language.
Why is Python good for blockchain?
Python programming language is the best to choose for blockchain implementation if you are a beginner. The main reason is that Python has comparatively shortcodes and is easier to use.
Building the Blockchain
This article provides a step-by-step guide to building a simple Python blockchain. So let’s get started.
How to implement blockchain in Python?
-
Instead of setting up any complicated development environment, we’ll simply go to Colaboratory, where you can easily write and execute code in Python
-
All you need to do is go to the website, log in using your Google account, and start writing Python code
-
Go to file and open a new notebook tab
First, import the Python blockchain library and create a block class along with a constructor.
import hashlib
class Block:
def _init_(self, data, prev_hash):
self.data = data
self.prev_hash = prev_hash
self.hash = self.calc_hash()
Now we’ll create a method that calculates the hash. For this, Python can use any standard cryptographic hash technique as mentioned in the US National Security Agency’s (NSA) set of SHA functions. As mentioned in the previous part, one of the major characteristics of blockchain is immutability which can be implemented using cryptography. This is a one-way algorithm that hashes the data to ensure security and privacy in the blockchain network. Here we will implement SHA-256 to compute the hash of the block.
def calc_hash(self):
sha = hashlib.sha256()
sha.update(self.data.encode('utf-8'))
return sha.hexdigest()
Now let’s create a blockchain class. To ensure immutability in entire blockchain networks, the blocks are interconnected with each other in such a way that the hash of the previous block is within the next block. This is how blockchain implementation in Python maintains integrity in the blockchain.
Here we initialize the blockchain and create the first block of the blockchain which is also known as the ‘Genesis Block’.
class Blockchain:
def _init_(self):
self.chain = [self.create_genesis_block()]
def create_genesis_block(self):
return Block("Genesis Block", "0")
In the next step, we’ll write code to add new blocks to the blockchain. Each new block has the hash value of the previous block. In this way, the blocks in the blockchain are connected to each other ensuring data security and controlled access.
def add_block(self, data):
prev_block = self.chain[-1]
new_block = Block(data, prev_block.hash)
self.chain.append(new_block)
Now let’s test the blockchain we’ve built. The code below prints the hash value of the current block and previous block with which you can see if your blockchain is giving the right output or not.
Blockchain = Blockchain()
blockchain.add_block('First block')
blockchain.add_block('Second block')
blockchain.add_block('Third block')
print('Blockchain:")
for block in blockchain.chain:
print('Data:', block.data)
print('Previous hash:', block.prev_hash)
print('Hash:', block.hash)
print()
Congratulations! You just built a simple Blockchain in Python from scratch.
Hire a Python Developer!
Build foolproof Python blockchain projects with our agile and high-grade Python developers. Partner with InvoBlox’s well-versed team of Python full-stack developers who are committed to your project’s success from the ground up.
Hire a Python Developer
In Python blockchain development, you can implement more functions like consensus method (proof-of-work or any other to define rules by which enhanced security can be achieved) or mining to strengthen the immutability of your blockchain.
A proof is a random number which is hard to find unless you have a high performance machine and equipment in place that is running around the clock to compute values. Other than this, you can add other details such as fees/reward for miners to mine the block, public/private keys, etc. that scale-up the functionality of your blockchain.